Interview Question: Tell one scenario where C# interface is indispensable. For the scenario, only C# interface is the solution and abstract class cannot be used.
Answer: Scenario is multiple interface inheritance. C# object oriented programming does not support multiple class inheritance, means, a class cannot inherit more than one class in C#. But, a class can inherit multiple interfaces.
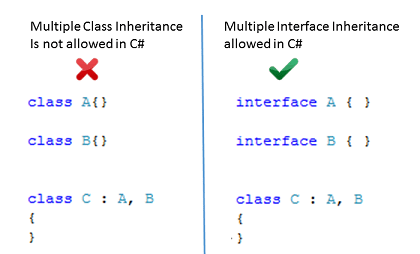
Multiple interface inheritance example in C#
//Create Ist interface
interface ICreditCard
{
void validateCard();
}
//Create 2nd interface
interface ITransaction
{
void startTransaction();
}
//Mastor credit card class will inherit multiple interfaces
class MastroCreditCard : ICreditCard, ITransaction
{
public void validateCard()
{
Console.WriteLine("Validating Credit Card");
}
public void startTransaction()
{
Console.WriteLine("Starting transaction");
}
public void transactionStatus()
{
Console.WriteLine("Transaction completed");
}
}
//Test
class Program
{
static void Main(string[] args)
{
MastroCreditCard card = new MastroCreditCard();
card.validateCard();
card.startTransaction();
card.transactionStatus();
}
}
Output:
Validating Credit Card
Starting transaction
Transaction completed
So, if a class has a requirement of multiple inheritance in C#, then only interfaces are solution not an abstract class.
Recommended another nice interview question here: what would you prefer between an interface and an abstract class in C# if both offer contracts only.